Picture by Writer
Constructing easy tasks is a good way to study Python and any programming language typically. You may study the syntax to write down for loops, use built-in features, learn recordsdata, and far more. However it’s solely whenever you begin constructing one thing that you simply truly “learn”.
Following the “learning by building” method, let’s code a easy TO-DO record app that we will run on the command line. Alongside the way in which we’ll discover ideas like parsing command-line arguments and dealing with recordsdata and file paths. We’ll as nicely revisit fundamentals like defining customized features.
So let’s get began!
By coding alongside to this tutorial, you’ll be capable of construct a TO-DO record app you’ll be able to run on the command line. Okay, so what would you just like the app to do?
Like TO-DO lists on paper, you want to have the ability to add duties, search for all duties, and take away duties (yeah, strikethrough or mark them completed on paper) after you’ve accomplished them, sure? So we’ll construct an app that lets us do the next.
Add duties to the record:
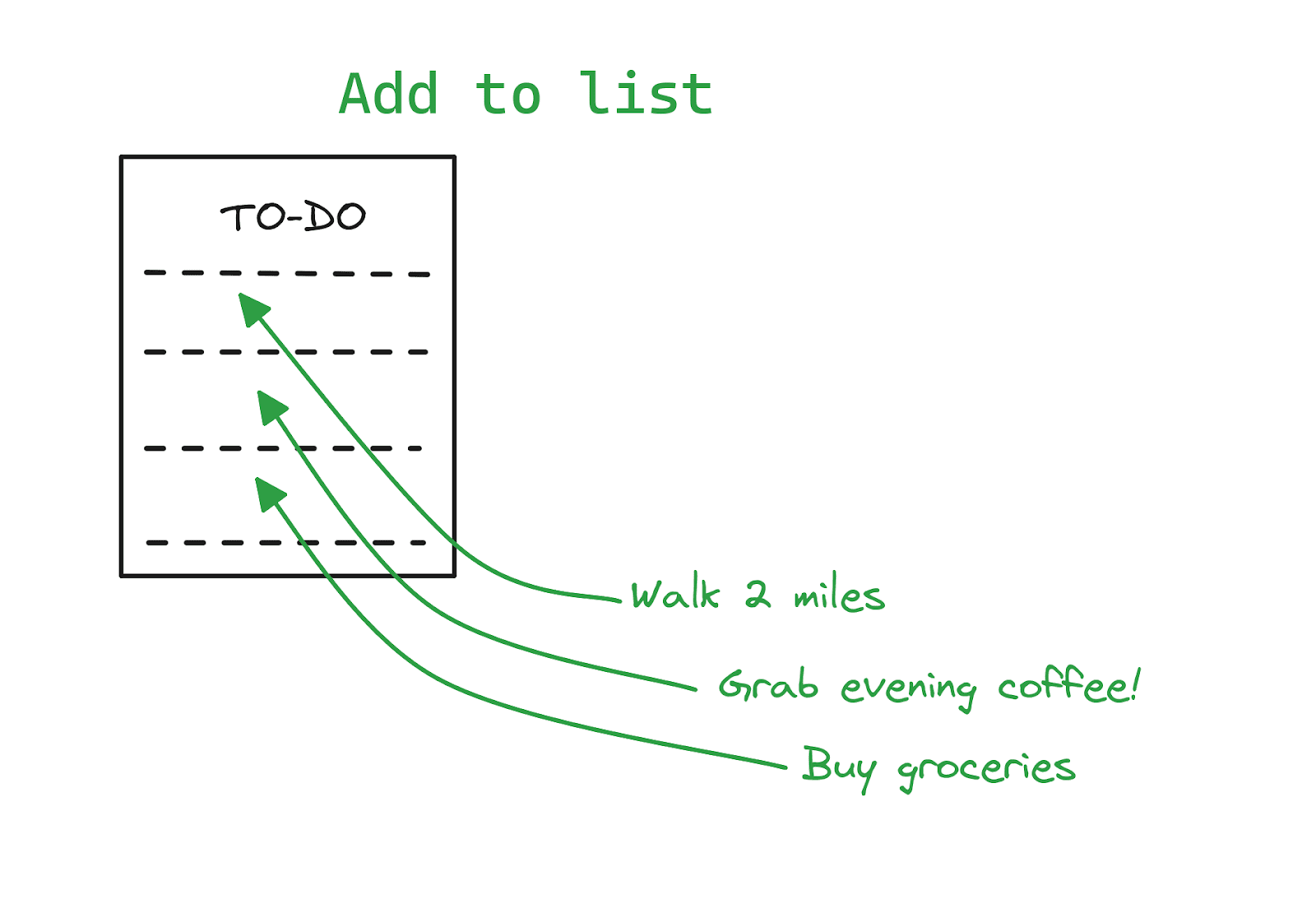
Picture by Writer
Get a listing of all duties on the record:
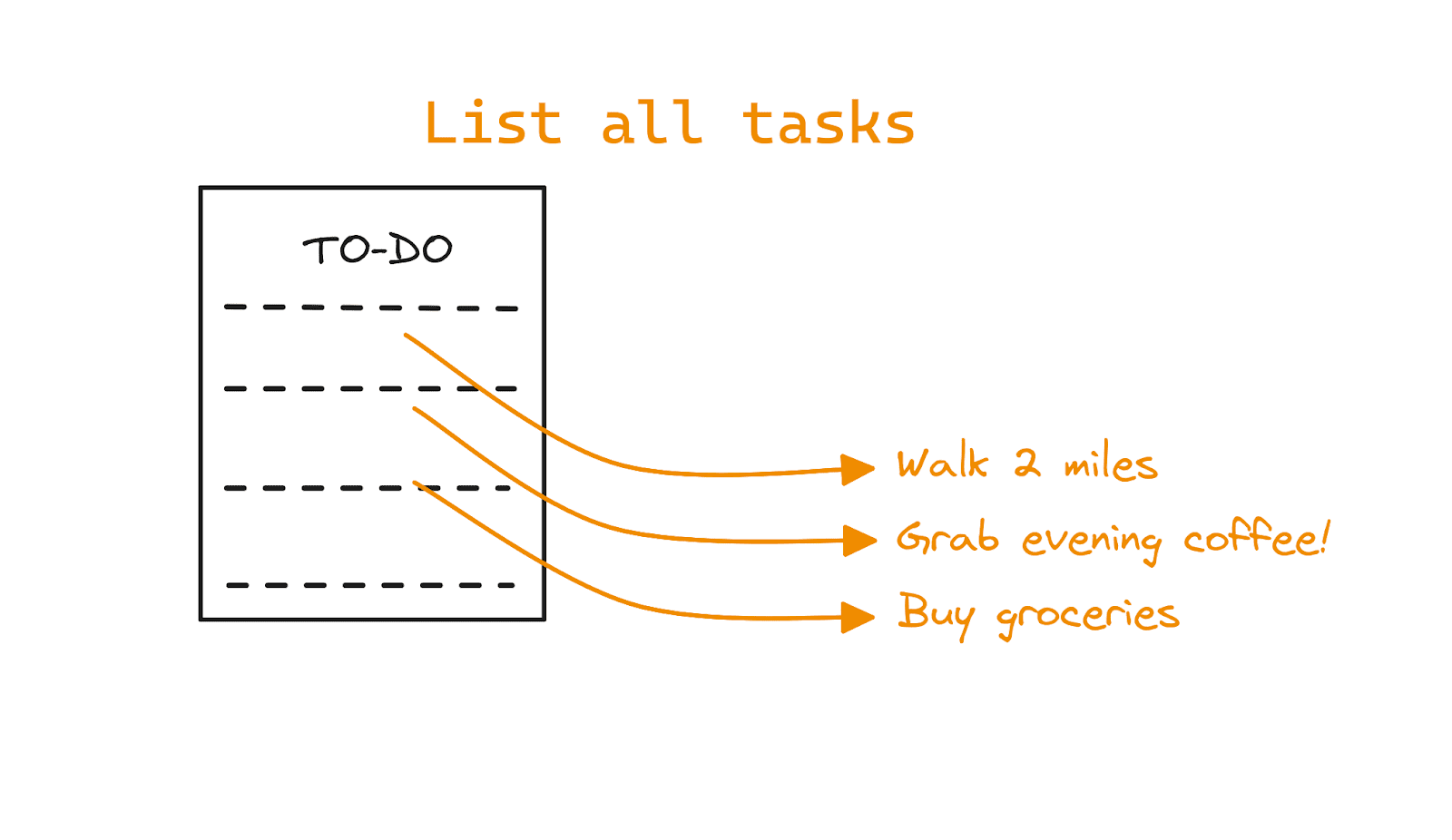
Picture by Writer
And in addition take away a job (utilizing its index) after you’ve completed it:
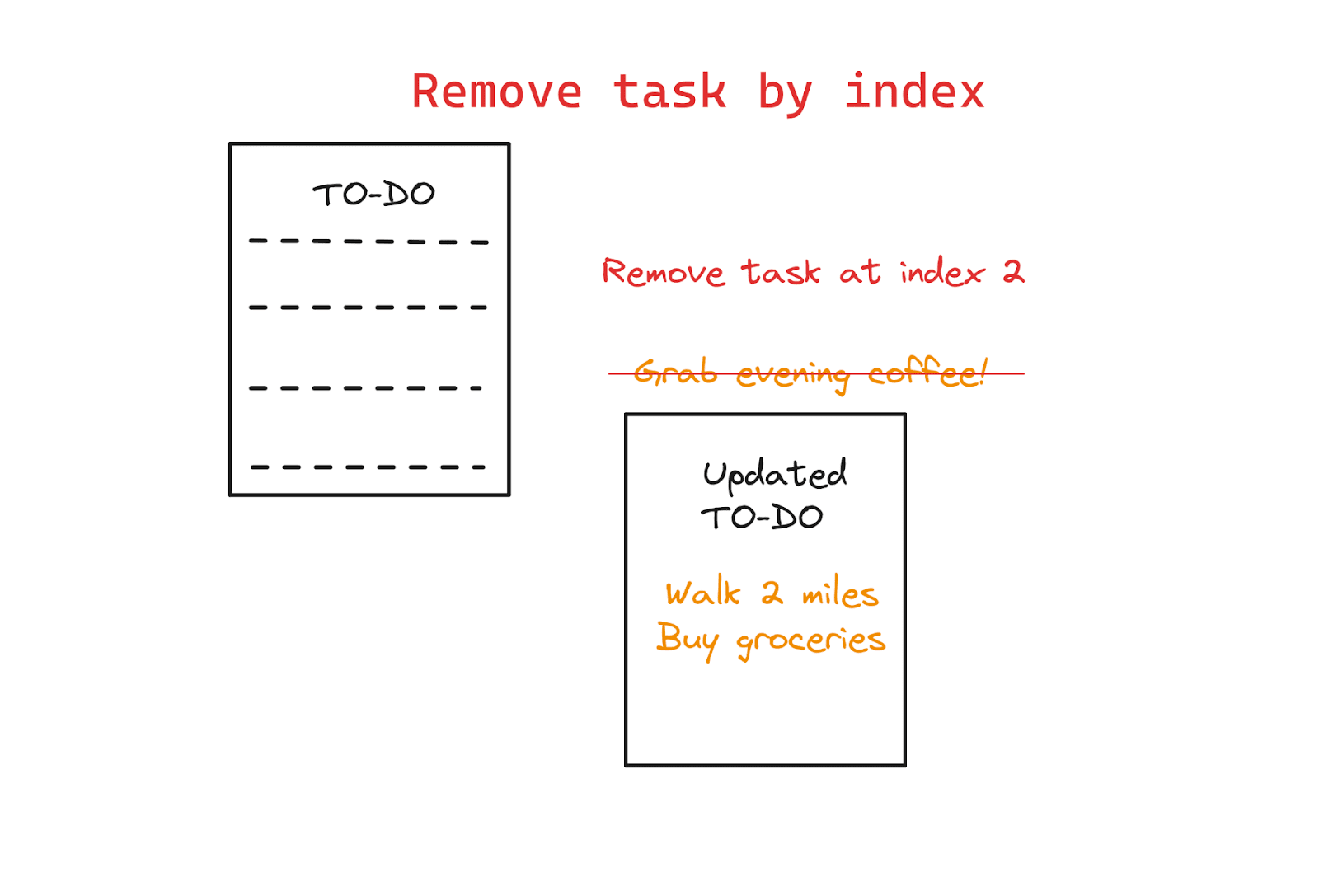
Picture by Writer
Now let’s begin coding!
First, create a listing on your mission. And contained in the mission listing, create a Python script file. This would be the primary file for our to-do record app. Let’s name it todo.py
.
You do not want any third-party libraries for this mission. So solely be sure to’re utilizing a latest model of Python. This tutorial makes use of Python 3.11.
Within the todo.py
file, begin by importing the required modules. For our easy to-do record app, we’ll want the next:
So let’s import them each:
import argparse
import os
Recall that we’ll use command-line flags so as to add, record, and take away duties. We are able to use each quick and lengthy choices for every argument. For our app, let’s use the next:
-a
or--add
so as to add duties-l
or--list
to record all duties-r
or--remove
to take away duties utilizing index
Right here’s the place we’ll use the argparse module to parse the arguments supplied on the command line. We outline the create_parser()
operate that does the next:
- Initializes an
ArgumentParser
object (let’s name itparser
). - Provides arguments for including, itemizing, and eradicating duties by calling the
add_argument()
technique on the parser object.
When including arguments we add each the quick and lengthy choices in addition to the corresponding assist message. So right here’s the create_parser()
operate:
def create_parser():
parser = argparse.ArgumentParser(description="Command-line Todo List App")
parser.add_argument("-a", "--add", metavar="", assist="Add a new task")
parser.add_argument("-l", "--list", motion="store_true", assist="List all tasks")
parser.add_argument("-r", "--remove", metavar="", assist="Remove a task by index")
return parser
We now have to outline features to carry out the next job administration operations:
- Including a job
- Itemizing all duties
- Eradicating a job by its index
The next operate add_task
interacts with a easy textual content file to handle objects on the TO-DO record. It opens the file within the ‘append’ mode and provides the duty to the top of the record:
def add_task(job):
with open("tasks.txt", "a") as file:
file.write(job + "n")
Discover how we’ve used the with
assertion to handle the file. Doing so ensures that the file is closed after the operation—even when there’s an error—minimizing useful resource leaks.
To study extra, learn the part on context managers for environment friendly useful resource dealing with in this tutorial on writing environment friendly Python code.
The list_tasks
operate lists all of the duties by checking if the file exists. The file is created solely whenever you add the primary job. We first examine if the file exists after which learn and print out the duties. If there are at present no duties, we get a useful message. :
def list_tasks():
if os.path.exists("tasks.txt"):
with open("tasks.txt", "r") as file:
duties = file.readlines()
for index, job in enumerate(duties, begin=1):
print(f"{index}. {task.strip()}")
else:
print("No tasks found.")
We additionally implement a remove_task
operate to take away duties by index. Opening the file within the ‘write’ mode overwrites the present file. So we take away the duty akin to the index and write the up to date TO-DO record to the file:
def remove_task(index):
if os.path.exists("tasks.txt"):
with open("tasks.txt", "r") as file:
duties = file.readlines()
with open("tasks.txt", "w") as file:
for i, job in enumerate(duties, begin=1):
if i != index:
file.write(job)
print("Task removed successfully.")
else:
print("No tasks found.")
We’ve arrange the parser to parse command-line arguments. And we’ve additionally outlined the features to carry out the duties of including, itemizing, and eradicating duties. So what’s subsequent?
You in all probability guessed it. We solely have to name the right operate primarily based on the command-line argument obtained. Let’s outline a primary()
operate to parse the command-line arguments utilizing the ArgumentParser
object we’ve created in step 3.
Primarily based on the supplied arguments, name the suitable job administration features. This may be completed utilizing a easy if-elif-else ladder like so:
def primary():
parser = create_parser()
args = parser.parse_args()
if args.add:
add_task(args.add)
elif args.record:
list_tasks()
elif args.take away:
remove_task(int(args.take away))
else:
parser.print_help()
if __name__ == "__main__":
primary()
Now you can run the TO-DO record app from the command line. Use the quick possibility h
or the lengthy possibility assist
to get info on the utilization:
$ python3 todo.py --help
utilization: todo.py [-h] [-a] [-l] [-r]
Command-line Todo Listing App
choices:
-h, --help present this assist message and exit
-a , --add Add a brand new job
-l, --list Listing all duties
-r , --remove Take away a job by index
Initially, there aren’t any duties within the record, so utilizing --list
to record all duties print out “No tasks found.”:
$ python3 todo.py --list
No duties discovered.
Now we add an merchandise to the TO-DO record like so:
$ python3 todo.py -a "Walk 2 miles"
If you record the objects now, you must be capable of see the duty added:
$ python3 todo.py --list
1. Stroll 2 miles
As a result of we’ve added the primary merchandise the duties.txt file has been created (Consult with the definition of the list_tasks
operate in step 4):
Let’s add one other job to the record:
$ python3 todo.py -a "Grab evening coffee!"
And one other:
$ python3 todo.py -a "Buy groceries"
And now let’s get the record of all duties:
$ python3 todo.py -l
1. Stroll 2 miles
2. Seize night espresso!
3. Purchase groceries
Now let’s take away a job by its index. Say we’re completed with night espresso (and hopefully for the day), so we take away it as proven:
$ python3 todo.py -r 2
Job eliminated efficiently.
The modified TO-DO record is as follows:
$ python3 todo.py --list
1. Stroll 2 miles
2. Purchase groceries
Okay, the best model of our app is prepared. So how can we take this additional? Right here are some things you’ll be able to attempt:
- What occurs whenever you use an invalid command-line possibility (say
-w
or--wrong
)? The default conduct (in the event you recall from the if-elif-else ladder) is to print out the assistance message however there’ll be an exception, too. Strive implementing error dealing with utilizing try-except blocks. - Check your app by defining check instances that embrace edge instances. To start out, you need to use the built-in unittest module.
- Enhance the present model by including an choice to specify the precedence for every job. Additionally attempt to type and retrieve duties by precedence.
▶️ The code for this tutorial is on GitHub.
On this tutorial, we constructed a easy command-line TO-DO record app. In doing so, we realized the best way to use the built-in argparse module to parse command-line arguments. We additionally used the command-line inputs to carry out corresponding operations on a easy textual content file beneath the hood.
So the place can we go subsequent? Effectively, Python libraries like Typer make constructing command-line apps a breeze. And we’ll construct one utilizing Typer in an upcoming Python tutorial. Till then, hold coding!
Bala Priya C is a developer and technical author from India. She likes working on the intersection of math, programming, information science, and content material creation. Her areas of curiosity and experience embrace DevOps, information science, and pure language processing. She enjoys studying, writing, coding, and low! At the moment, she’s engaged on studying and sharing her data with the developer group by authoring tutorials, how-to guides, opinion items, and extra. Bala additionally creates partaking useful resource overviews and coding tutorials.